1. Show one piece of data through a label.
2. Have a Next and Previous button.
Like this:
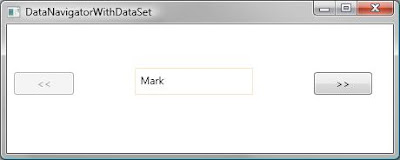
Pretty simple window. Very hard to figure out. It took A LOT of study. Frankly I was surprised I couldn't find a clean cut answer anywhere for how to do this...hence, the following post.
The basic steps:
1. Add a class variable to store the CollectionView (tracks current record being displayed).
2. Set this.DataContext to your data source.
3. Set the variable you created in step one to
(CollectionView)
CollectionViewSource.GetDefaultView(DataContext)
4. Add event handling for your variable's CollectionView.CurrentChanged event.
Here's the XAML:
1: <Window x:Class="DataBindingExample.DataNavigatorWithDataSet"
2: xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
3: xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
4: Title="DataNavigatorWithDataSet" Height="190" Width="460">
5: <Grid>
6: <Button HorizontalAlignment="Right" Margin="0,55,24,67" Width="67" Content=">>" x:Name="btnNext" Click="btnNext_Click" />
7: <Button HorizontalAlignment="Left" Margin="8,55,0,67" Width="69" Content="<<" x:Name="btnPrevious" Click="btnPrevious_Click" />
8: <Label Content="{Binding Path=FirstName}" Margin="147.407,50,160.747,67" Name="label1" BorderBrush="Bisque" BorderThickness="1"></Label>
9: </Grid>
10: </Window>
1: using System.Windows;
2: using System.Windows.Data;
3:
4: namespace DataBindingExample
5: {
6: public partial class DataNavigatorWithDataSet : Window
7: {
8: private CollectionView view;
9:
10: public DataNavigatorWithDataSet()
11: {
12: InitializeComponent();
13: PopulatePeople();
14: }
15:
16: private void PopulatePeople()
17: {
18: Data data = new Data();
19: DataContext = data.GetPeopleDataSet().PersonDataTable;
20: view = (CollectionView)CollectionViewSource.GetDefaultView(DataContext);
21:
22: view.CurrentChanged += delegate
23: {
24: btnPrevious.IsEnabled = (view.CurrentPosition > 0);
25: btnNext.IsEnabled = (view.CurrentPosition < view.Count - 1);
26: };
27: }
28:
29: private void btnNext_Click(object sender, RoutedEventArgs e)
30: {
31: view.MoveCurrentToNext();
32: }
33:
34: private void btnPrevious_Click(object sender, RoutedEventArgs e)
35: {
36: view.MoveCurrentToPrevious();
37: }
38: }
39: }