Look at the example below. The Border element has its style defined inline. I define a Style just for my Border elements based on its existing properties:
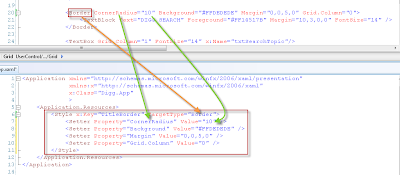
<Border Style="{StaticResource TitleBorder}">
Hi, I'm Mark Moeykens. I created this blog so I could post and reference my Windows Presentation Foundation info in one central location. Since I'm thinking I'm the only one that will be referencing this blog, I warn you to use the info at your own risk! Sorry, I might omit data if I already know it.
<Border Style="{StaticResource TitleBorder}">
Content
property that means that you can put another control inside that control. It is somewhat of a container but can only support ONE control. So you could do this:
<Label Height="Auto" VerticalAlignment="Top"> <StackPanel> <Border BorderBrush="Black" CornerRadius="2,2,2,2"> <TextBlock>Label with text and border</TextBlock> </Border> </StackPanel> </Label>But you can't do this:
public static DependencyProperty ConditionProperty;2. Create a regular property with a get and set. This is usually the same name as your DependencyProperty but without the suffix "Property".
public enum ConditionEnum { NonExistence, Danger, Emergency, Normal, Affluence, Power } public ConditionEnum Condition { get { return (ConditionEnum)GetValue(ConditionProperty); } set { SetValue(ConditionProperty, value); } }(I'm using an enum as the type for this property.)
private static void Condition_Changed(DependencyObject sender, DependencyPropertyChangedEventArgs e) { ConditionEnum conditionValue = (ConditionEnum) e.NewValue; StatGraphControl thisUserControl = (StatGraphControl) sender; thisUserControl.BackgroundRectangle.Fill = new SolidColorBrush((Color) thisUserControl.FindResource(conditionValue.ToString())); }Notice how you get the value of what the property is being set to. You also have to get the user control itself from the sender parameter if you are going to change something on your user control. All properties and objects cannot be referenced directly from the same class because you are working with a static context. So you have to work on the properties and objects of the control passed in from the sender parameter.
<UserControl.Resources> <Color x:Key="NonExistence">#FF808080</Color> <Color x:Key="Danger">#FFBE0000</Color> <Color x:Key="Emergency">#FFFF8D07</Color> <Color x:Key="Normal">#FFF0C908</Color> <Color x:Key="Affluence">#FF1AA451</Color> <Color x:Key="Power">#FF166C0D</Color> </UserControl.Resources>4. Create a static constructor to register your dependency property with your property and event. This last step kind of ties everything together.
1: static StatGraphControl()
2: {
3: ConditionProperty = DependencyProperty.Register(
4: "Condition",
5: typeof(ConditionEnum),
6: typeof(StatGraphControl),
7: new PropertyMetadata(
8: ConditionEnum.NonExistence,
9: Condition_Changed));
10: }
Category
attribute to your public property. There is also a Description
attribute you can add if you think it will help.
[Category("MyApp")] [Description("Setting the Condition will determine what color shows.")] public ConditionEnum Condition { get { return (ConditionEnum)GetValue(ConditionProperty); } set { SetValue(ConditionProperty, value); } }
(CollectionView)
CollectionViewSource.GetDefaultView(DataContext)
1: <Window x:Class="DataBindingExample.DataNavigatorWithDataSet"
2: xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
3: xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
4: Title="DataNavigatorWithDataSet" Height="190" Width="460">
5: <Grid>
6: <Button HorizontalAlignment="Right" Margin="0,55,24,67" Width="67" Content=">>" x:Name="btnNext" Click="btnNext_Click" />
7: <Button HorizontalAlignment="Left" Margin="8,55,0,67" Width="69" Content="<<" x:Name="btnPrevious" Click="btnPrevious_Click" />
8: <Label Content="{Binding Path=FirstName}" Margin="147.407,50,160.747,67" Name="label1" BorderBrush="Bisque" BorderThickness="1"></Label>
9: </Grid>
10: </Window>
1: using System.Windows;
2: using System.Windows.Data;
3:
4: namespace DataBindingExample
5: {
6: public partial class DataNavigatorWithDataSet : Window
7: {
8: private CollectionView view;
9:
10: public DataNavigatorWithDataSet()
11: {
12: InitializeComponent();
13: PopulatePeople();
14: }
15:
16: private void PopulatePeople()
17: {
18: Data data = new Data();
19: DataContext = data.GetPeopleDataSet().PersonDataTable;
20: view = (CollectionView)CollectionViewSource.GetDefaultView(DataContext);
21:
22: view.CurrentChanged += delegate
23: {
24: btnPrevious.IsEnabled = (view.CurrentPosition > 0);
25: btnNext.IsEnabled = (view.CurrentPosition < view.Count - 1);
26: };
27: }
28:
29: private void btnNext_Click(object sender, RoutedEventArgs e)
30: {
31: view.MoveCurrentToNext();
32: }
33:
34: private void btnPrevious_Click(object sender, RoutedEventArgs e)
35: {
36: view.MoveCurrentToPrevious();
37: }
38: }
39: }
<TextBlock FontSize="{Binding ElementName=sliderFontSize, Path=Value}" />You will change "ElementName" to one of the following:
public class Person { public string FirstName { get; set; } public string LastName { get; set; } }2. Create your Bindings
<Grid Name="gridPerson"> <TextBox Text="{Binding Path=FirstName}" /> <TextBox Text="{Binding Path=LastName}" /> </Grid>Note: The field name used for Path is CASE SENSITIVE. Your application will not generate a build error either. If your data is not showing up, check the case of the field name.
gridPerson.DataContext = App.Database.GetPerson();Note:
DataContext = App.Database.GetPerson();is valid also. Using gridPerson.DataContext just narrows the scope and makes the data only available to elements within gridPerson.
<Slider Name="sliderFontSize" Minimum="1" Maximum="40" Value="10" TickFrequency="1" TickPlacement="TopLeft" /> <TextBlock Name="lblSampleText" Text="Simple Text" FontSize="{Binding Source=sliderFontSize, Path=Value}" />The TextBlock's FontSize will change to the slider control's value. This binding in the xaml is saying, "Create a binding to the sliderFontSize.Value property."
Binding binding = new Binding(); binding.Source = sliderFontSize; binding.Path = new PropertyPath("Value"); lblSampleText.SetBinding(TextBlock.FontSizeProperty, binding);More Info: Data Binding